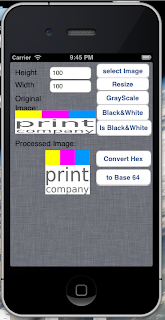
here, we allow the user to enter a preferred height and width for the image to be re sized. Once done and click on the re size button, the re sized image will be displayed in the UIImageView below. Following is the implementation
//method to resize the image
//user needs to input the required height and width in the screen
//and cick Resize to apply the resize funtion
-(IBAction) resize:(id)sender
{
[self.view endEditing:YES];
if(sourceImage==nil)
{
}
else
{
if([getWidth.text isEqualToString:@""] || [getHeight.text isEqualToString:@""])
{
alert=[[UIAlertView alloc]initWithTitle:@"Image Process" message:@"Height & Width Required" delegate:nil cancelButtonTitle:@"Cancel" otherButtonTitles:@"OK", nil];
[alert show];
}
else
{
CGFloat destinationWidth = [getWidth.text floatValue];
CGFloat destinationHeight =[getHeight.text floatValue];
CGFloat xOfResultImage = (CGFloat)resultImageView.frame.origin.x;
CGFloat yOfResultImage = (CGFloat)resultImageView.frame.origin.y;
CGSize passSize=CGSizeMake(destinationWidth, destinationHeight);
UIImage *resizedImage=[self scaleImage:sourceImage toSize:passSize];
resultImageView.image=resizedImage;
resultImageView.frame = CGRectMake(xOfResultImage, yOfResultImage,resizedImage.size.width, resizedImage.size.height);
}
}
}
//to scale images without changing aspect ratio
- (UIImage *)scaleImage:(UIImage *)image toSize:(CGSize)newSize
{
float width = newSize.width;
float height = newSize.height;
UIGraphicsBeginImageContext(newSize);
CGRect rect = CGRectMake(0, 0, width, height);
float widthRatio = image.size.width / width;
float heightRatio = image.size.height / height;
float divisor = widthRatio > heightRatio ? widthRatio : heightRatio;
width = image.size.width / divisor;
height = image.size.height / divisor;
rect.size.width = width;
rect.size.height = height;
//indent in case of width or height difference
float offset = (width - height) / 2;
if (offset > 0)
{
rect.origin.y = offset;
}
else
{
rect.origin.x = -offset;
}
[image drawInRect: rect];
UIImage *resizedImage = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
return resizedImage ;
}
No comments:
Post a Comment